Example of Algorithm in Real Life
Washing clothes
Analysis :
Problem : Algorithm of washing clothes with automatic washing machine
Requirement :
Input : Clothes, detergent, softener
Process : Put the clothes into the washing machine then washed
Output : Clothes washed and dried
Algorithm :
Plug the washing machine cable into the stop-contact
Open the washing machine's top
Put clothes into the machine
Add detergent and softener
Close the machine's top
Turn it on by press the power button
Set the water discharge
Select wash mode
Press start button
Wait until the washing is finished
When it's finished, turn off the machine
Unplug the cable
Open the top of the machine
Take out the clothes from the washing machine
Hang clothes under the sun
Flowchart
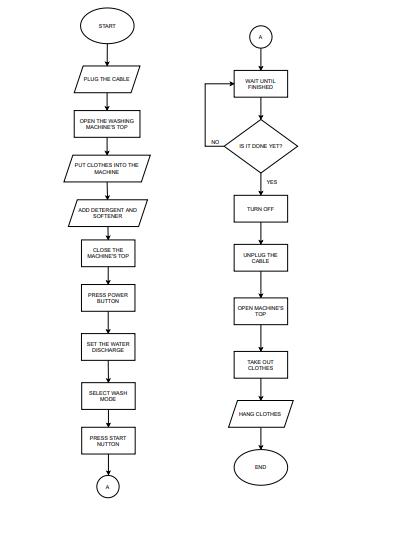
The Remaining Money for Groceries
Analysis :
Problem : Algorithm to find the total amount of change for groceries
Requirement :
Input : The number of types of goods purchased, the number of purchases per item, unit price, total of money payment
Process : Calculating the number of change based on the entered data
Output : Total amount of change
Algorithm :
Enter the number of types
Repeat the steps below for each item :
Enter the amount of money payment
Find the purchase price (buy_price <-- sum * each)
Find the total purchase price (total += buy_price)
Find change (change = pay - total)
C++ Program :
#include <iostream>
using namespace std;
struct change{
string name;
int sum;
int each;
}buy[100];
int main (){
int n, i, pay, change;
int buy_price[100];
int total = 0;
cout<<"Enter the amount of types : ";
cin>>n;
cout<<endl;
for (i=1;i<=n;i++){
cout<<"Item-"<<i<<" : ";
cin>>buy[i].name;
cout<<"Sum of "<<buy[i].name<<" = ";
cin>>buy[i].sum;
cout<<"Price per each "<<buy[i].name<<" = ";
cin>>buy[i].each;
cout<<endl;
}
cout<<"Enter the amount of money payment : ";
cin>>pay;
cout<<endl;
for (i=1;i<=n;i++){
buy_price[i]=buy[i].sum*buy[i].each;
cout<<"Price of "<<buy[i].name<<" = "<<buy[i].sum<<" * "<<buy[i].each<<" = "<<buy_price[i];
cout<<endl;
}
cout<<endl;
for (i=1;i<=n;i++){
total+=buy_price[i];
}
cout<<"Price total = "<<total;
cout<<endl;
change=pay-total;
cout<<"Sum of change = "<<change;
return 0;
}
Output :

Comments