C ++ Program and Algorithm | Checks Whether The Input Character is Uppercase, Lowercase, or Digits
- D A Rosi Arsida Wardani
- May 1, 2021
- 1 min read
Algorithm character_check
{checks whether the input character is uppercase, lowercase, or digits}
Declaration
character : char
Description
read (character)
if (character >= 25 And character <= 90) then
write (Uppercase)
else if (character >= 97 And character <= 122) then
write (Lowercase)
else if (character >= 47 And character <= 57) then
write (Digit)
Flowchart
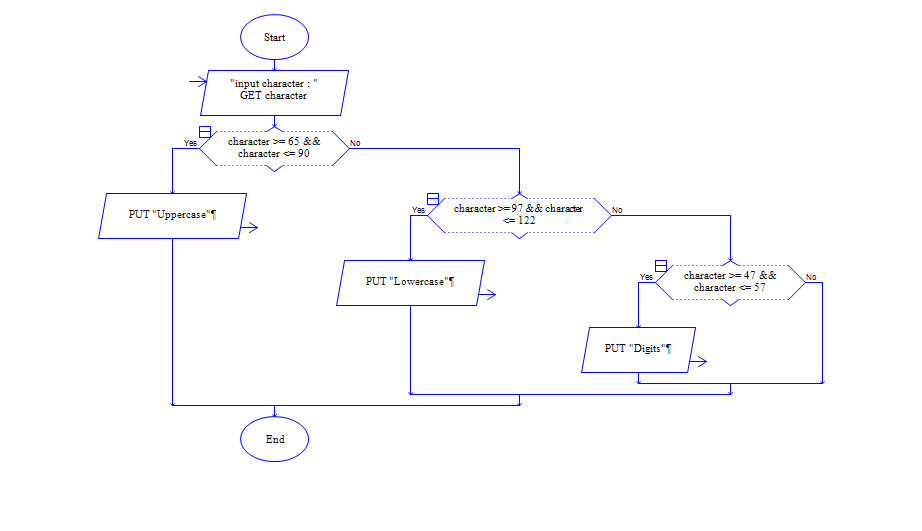
Program C++
#include <iostream>
using namespace std;
class character_check{
friend ostream& operator<<(ostream&, character_check&);
friend istream& operator>>(istream&, character_check&);
public :
char character;
void process();
};
istream& operator>>(istream& in, character_check& input){
cout<<"input character : ";
in>>input.character;
return in;
}
void character_check::process(){
if (character >= 65 && character <= 90){
cout<<"Uppercase";
}else if (character >= 97 && character <= 122){
cout<<"Lowercase";
}else if (character >= 47 && character <= 57){
cout<<"Digits";
}
}
ostream& operator << (ostream& out, character_check& outp){
outp.process();
return out;
}
int main(){
character_check x;
cin>>x;
cout<<x;
}
Output :
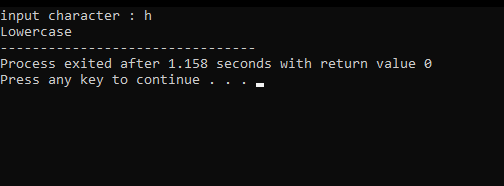
Comments