C ++ Program and Algorithm | Separate integer numbers less than 1000 into their components
- D A Rosi Arsida Wardani
- Apr 25, 2021
- 1 min read
Analysis :
To get the number hundreds, the formula is :
hundreds = number/100
To get the number tens, the formula is :
tens = (number % 100) / 10
Meanwhile, to get the units number, the formula is :
units = (number % 100) % 10
Algorithm integer_components :
{separate integer numbers less than 1000 into their components}
Declaration :
num, hundreds, tens, units : integer
components : string
Description :
read (num)
if (num>=100) then
hundreds ← num/100
tens ← (num%100)/10
units ← (num%100)%10
write (components)
else if (num >= 10 And num<=99) then
tens ← (num%100)/10
units ← (num%100)%10
write (components)
else
units ← (num%100)%10
write (components)
endif
Flowchart :
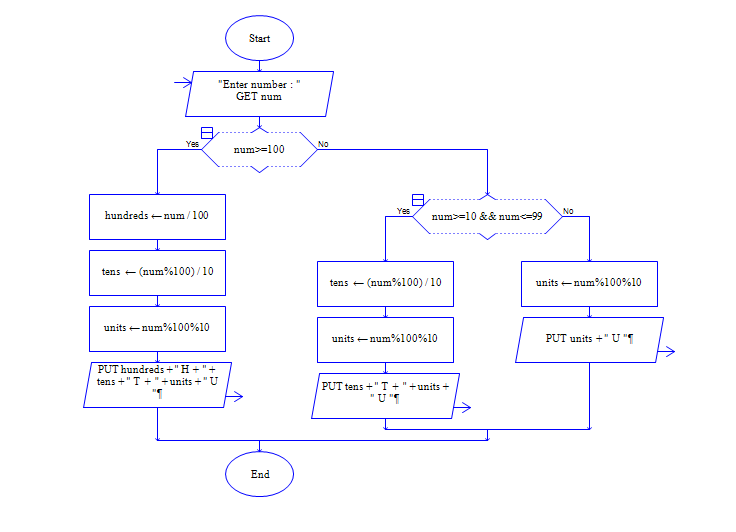
Here's the output if we input 167 :

C++ Program :
#include <iostream>
using namespace std;
int main(int argc, char** argv){
int num, hundreds, tens , units;
cout<<"Enter number : ";
cin>>num;
if(num >=100){
hundreds = num / 100;
tens = (num%100)/10;
units = (num % 100) % 10;
cout<<hundreds<<" H + "<<tens<<" T + "<<units<<" U ";
} else if(num >=10 && num <=99){
tens = (num%100)/10;
units = (num % 100) % 10;
cout<<tens<<" T + "<<units<<" U ";
} else {
units = (num % 100) % 10;
cout<<units<<" U ";
}
return 0;
}
Output :

Comments